How to Use the crypto npm Module in Node.js for Cryptographic Functionality
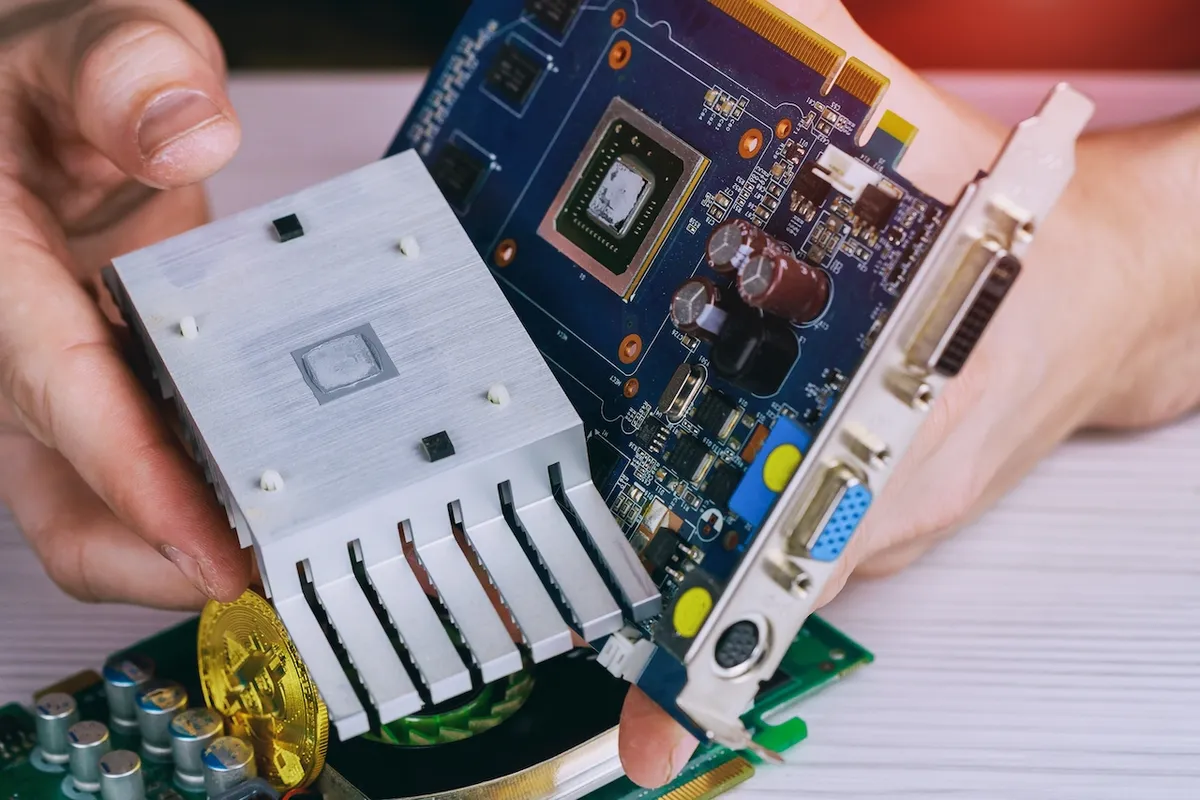
Securely managing information is a top concern for developers in the digital world. The crypto module is Node.js's built-in library that offers powerful tools to safeguard data. Our guide will navigate you through using this module, equipping you with the ability to encrypt, decrypt, and hash data effortlessly.
Stay safe in the code jungle – let's get started!
Understanding the crypto module in Node. js
The crypto module in Node.js provides cryptographic functionality for secure communication and data protection. It includes various encryption, decryption, hashing, and digital signature algorithms for implementing secure applications.
Deprecated packages
Some old packages for Node.js are not safe to use anymore. This is because the people who made them no longer update or fix them. If you find an old package, it's best not to use it and look for something newer that does the same thing.
Next, let's talk about how to put the crypto module on your computer and start using it.
Installation
To use the crypto npm module in Node.js for cryptographic functionality, you can install it by running the following command in your Node.js project directory:
- Run the command `npm install crypto` to install the crypto module.
- Once installed, you can import the crypto module into your Node.js application using `const crypto = require('crypto');`.
- Ensure that your Node.js environment has npm installed locally by running `npm -v` and node by running `node -v`.
Usage
To use the crypto module in Node.js, begin by installing it using the npm command. Once installed, you can require it in your code to access various cryptographic functions such as encryption, hashing, digital signatures, and key management.
Ensure that you update to the latest version for enhanced security measures and follow best practices for securely storing and handling keys. When implementing cryptographic functionality in your Node.js applications, always prioritize secure communication over networks to maintain the integrity of your data.
After understanding the crypto module's capabilities and installing it in your Node.js environment, you can seamlessly integrate powerful cryptographic functions into your applications.
Available Cryptographic Functions
Explore the various cryptographic functions available in the crypto npm module, including encryption (AES, ECIES), hashing (SHA256), and signing and verifying data. Learn how these functions can be implemented for secure communication and data protection within Node.js applications.
Encryption (AES, ECIES)
The crypto npm module in Node.js provides strong encryption capabilities, including AES (Advanced Encryption Standard) and ECIES (Elliptic Curve Integrated Encryption Scheme). These encryption methods allow you to securely encode sensitive data, making it unreadable to unauthorized users.
By leveraging AES and ECIES within the crypto module, you can ensure that your Node.js applications are equipped with robust cryptographic functionality, enabling secure communication and data protection.
Implementing encryption using AES and ECIES involves generating keys for encrypting and decrypting data. With the crypto module in Node.js, you can easily incorporate these encryption techniques into your application, safeguarding sensitive information from unauthorized access.
Hashing (SHA256)
The SHA256 hashing function in the crypto module of Node.js generates a fixed-size string of characters from input data, making it ideal for creating secure digital signatures and verifying data integrity.
It produces a unique hash that is irreversible and consistent across different platforms. The SHA256 algorithm can help ensure the authenticity and integrity of sensitive information, such as passwords or message digests, by generating a hash that uniquely represents the input data.
Now let's dive into another critical aspect related to cryptographic functionality: "Signing and Verifying."
Signing and Verifying
After hashing data using SHA256, you can ensure its integrity by signing and verifying it. Signing involves creating a digital signature using a private key, while verifying uses the corresponding public key to confirm the authenticity of the signature.
This process helps in proving that the data has not been tampered with during transmission or storage.
In Node.js, you can achieve signing and verification using the crypto module's specific functions. The `crypto.createSign()` method is used for signing data, while `crypto.createVerify()` verifies the signature against the provided data.
How to Implement the Crypto Module
Implementing the crypto module involves generating keys, encrypting and decrypting data, as well as signing and verifying data. If you want to learn more about how to use these cryptographic functionalities in Node.js, keep reading!
Generating keys
To generate keys in the crypto module, you can use the `generateKeyPair` method to create a public and private key pair for asymmetric encryption. The following steps outline the process:
- Use the `crypto.generateKeyPairSync()` method to synchronously generate an asymmetric key pair.
- Specify the type of keys to be generated, such as 'rsa' or 'dsa', along with other parameters like modulusLength and namedCurve.
- Store the keys securely, ensuring that the private key is accessible only to authorized entities and not exposed.
Encrypting and decrypting data
After generating keys, the next step is encrypting and decrypting data. This process involves using cryptographic algorithms to convert plain text into cipher text and back again. Here’s a detailed look at how to accomplish this:
- Choose the appropriate encryption algorithm based on your security needs. AES (Advanced Encryption Standard) is commonly used for its strong encryption capabilities.
- Use the chosen algorithm along with the generated keys to encrypt sensitive data. This process will convert the plaintext into ciphertext, rendering it unreadable without the corresponding decryption key.
- When decrypting data, employ the same cryptographic algorithm and the decryption key to convert the ciphertext back into its original plaintext form.
- Always ensure that encrypted data is transmitted and stored securely to prevent unauthorized access or tampering.
- Remember to handle encryption and decryption keys with extreme care, as they are crucial for safeguarding sensitive information.
- Regularly review and update your encryption methods to align with industry best practices and address any emerging security threats.
Signing and verifying data
To ensure data integrity and authenticity, the crypto module in Node.js allows for signing and verifying data using digital signatures. Here's how to implement it:
- Generate a key pair - Use the crypto module to generate a public-private key pair for signing and verifying data.
- Signing data - Utilize the private key to create a digital signature for the data using cryptographic algorithms like RSA or ECDSA.
- Verifying data - Verify the integrity of the data by using the public key to authenticate the digital signature.
- Ensuring secure communication - Use signed messages to ensure secure communication over networks, providing assurance of data origin and integrity.
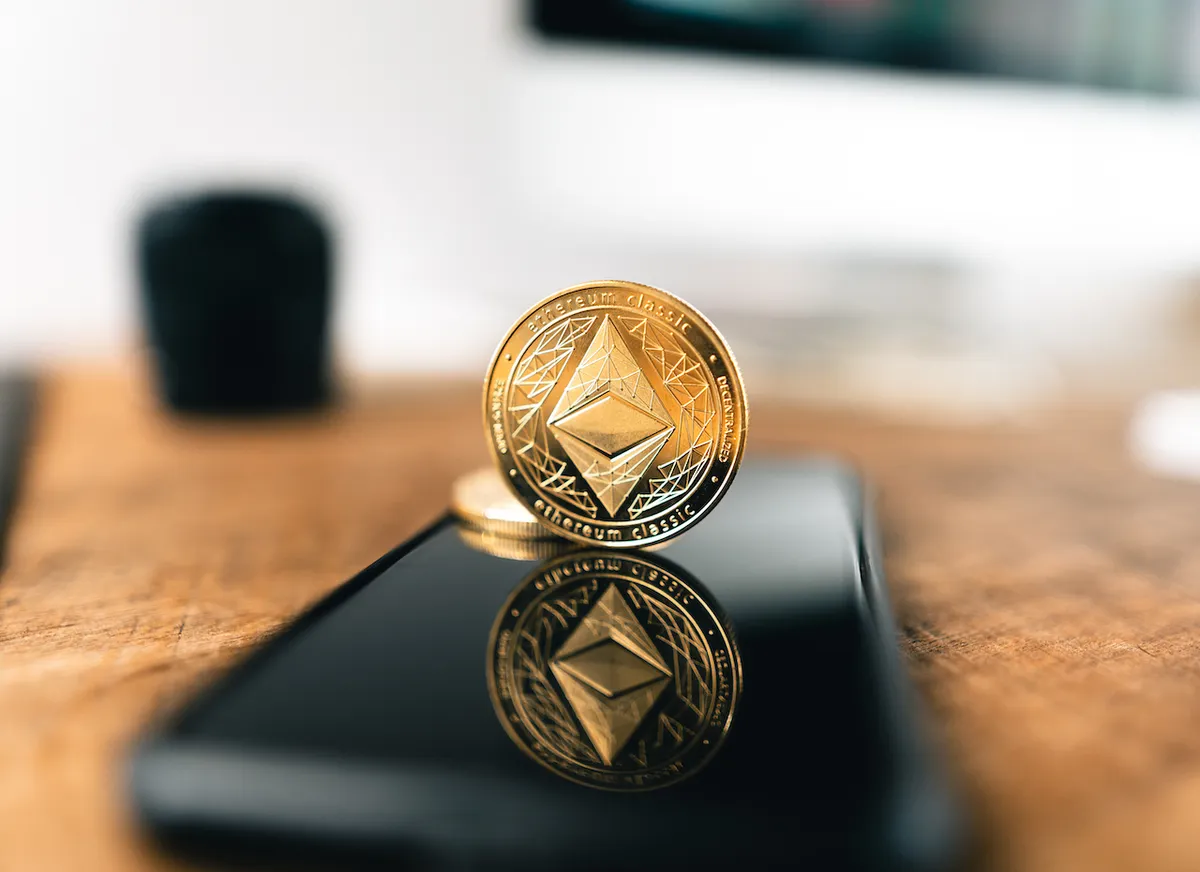
Best Practices for Using Crypto Module
Ensure to regularly update to the latest version of the crypto module, properly store and handle keys, and implement secure communication over networks for optimal cryptographic functionality in Node.js.
Updating to the latest version
To ensure the security and stability of your application, it is crucial to regularly update the crypto npm module in Node.js. By staying current with the latest version, you can benefit from important security patches, bug fixes, and enhanced cryptographic functionalities.
Additionally, updates often introduce performance improvements, ensuring that your cryptographic operations are executed efficiently and effectively. Keeping the crypto module up to date also helps in aligning your application with industry best practices for secure authentication and data protection.
It is essential to prioritize updating to the latest version of the crypto npm module in Node.js as part of your ongoing efforts to maintain a robust and secure authentication process within your Node.js applications.
Staying informed about new releases and promptly integrating them into your codebase ensures that you leverage the most advanced cryptographic features while mitigating potential vulnerabilities associated with outdated versions.
Properly storing and handling keys
To properly store and handle keys in the crypto module of Node.js:
- Use a secure key management system to store keys, such as a hardware security module (HSM) or a secure key vault.
- Avoid hardcoding or embedding keys directly within source code to prevent unauthorized access.
- Regularly rotate and update keys to mitigate the risk of potential breaches.
- Implement strong access controls and permissions to restrict key usage to authorized entities only.
- Encrypt keys at rest using robust encryption algorithms to protect them from unauthorized access.
- Follow established cryptographic best practices and standards when generating, storing, and handling keys.
Ensuring secure communication over networks
After properly storing and handling keys, it's crucial to ensure secure communication over networks when using the crypto module in Node.js. To achieve this, always use encrypted communication protocols such as HTTPS for web applications or SSL/TLS for network communication.
Additionally, implement proper authentication mechanisms and validate data input to prevent unauthorized access or tampering of sensitive information.
When transmitting data over networks, make sure to avoid hardcoding cryptographic keys within the source code and instead rely on secure key management systems. Regularly update encryption algorithms and follow security best practices recommended by experts in the field to stay ahead of potential vulnerabilities that could compromise network communication.
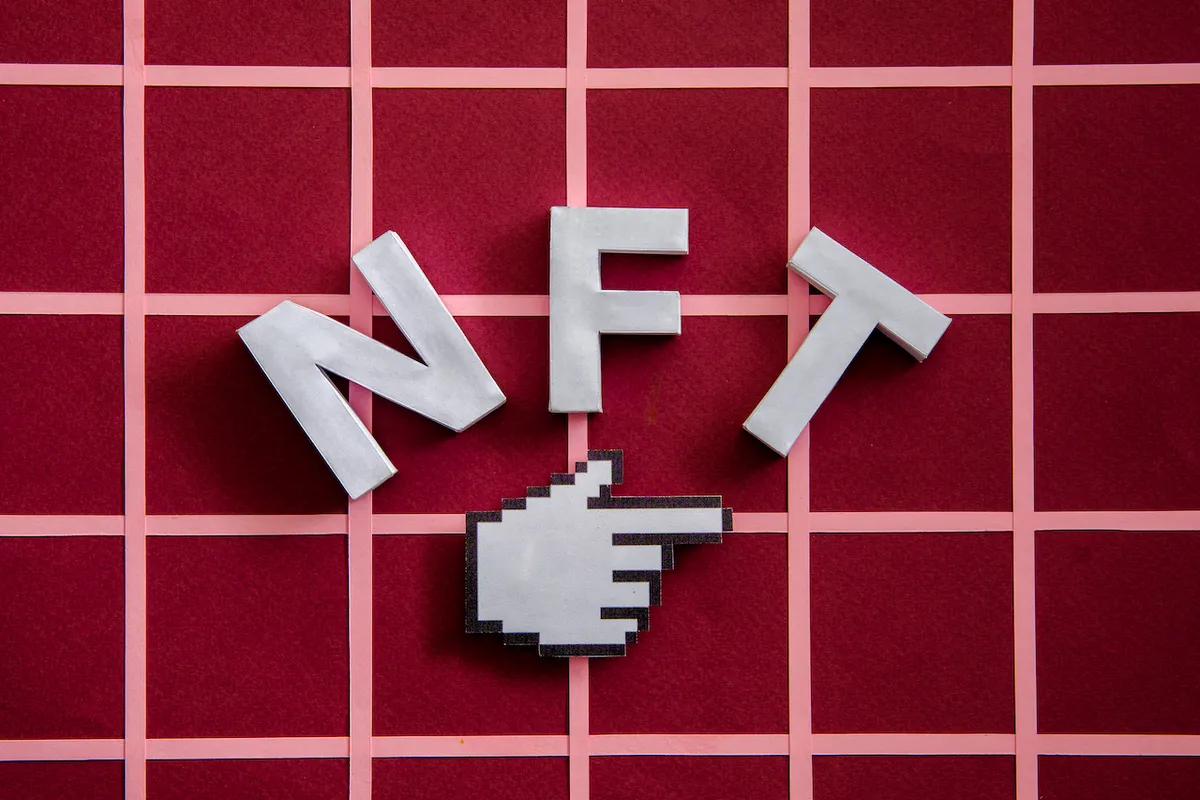
Conclusion
In conclusion, the crypto module in Node.js offers powerful cryptographic functions such as encryption, hashing, and signing. Implementing this module is straightforward and brings practical benefits to data security.
By following best practices such as updating to the latest version and properly handling keys, users can ensure a secure communication environment. Embracing these strategies will lead to improved data protection and set the stage for successful cryptographic operations in Node.js.
For further exploration of this topic, consider consulting official documentation or reputable online resources. Endeavor to apply these strategies effectively for enhanced cybersecurity in your projects.
Get legally married online
In partnership with Courtly, get legally married online.
From start to “I do.” Courtly team is fully committed to the cause: getting you married. They've done more remote weddings than anyone. Thousands of couples have counted on Courtly and we know what it takes to secure your legal marriage certificate.
Also, enjoy an exclusive discount available only to the MarryOnChain community! Use code MARRYONCHAIN to get $75 off your wedding.
RelatedRelated articles
All posts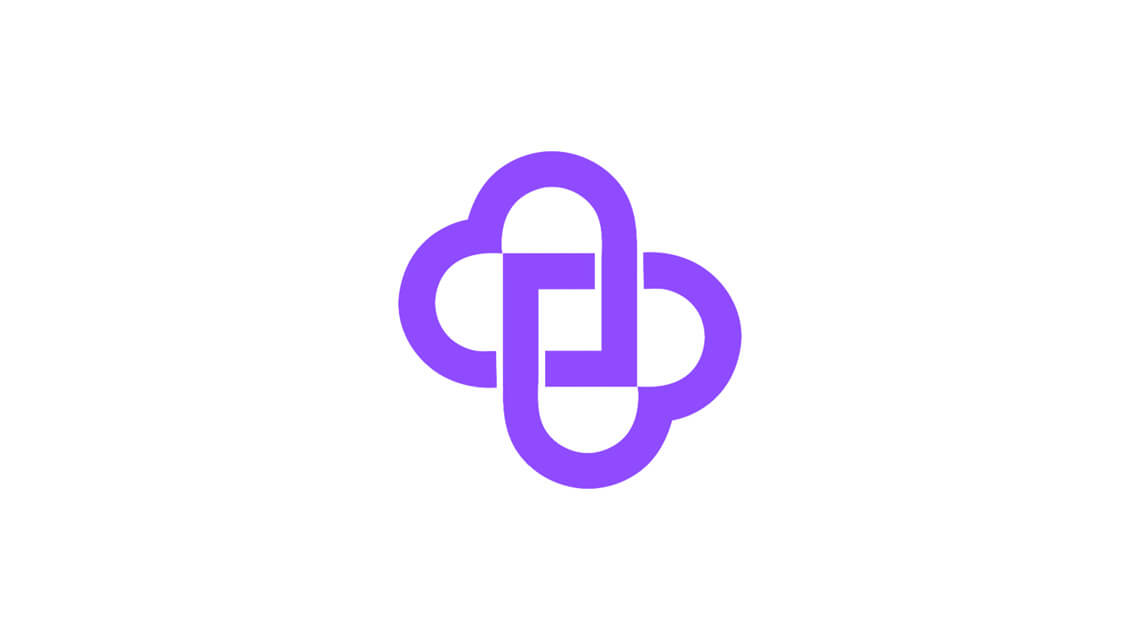
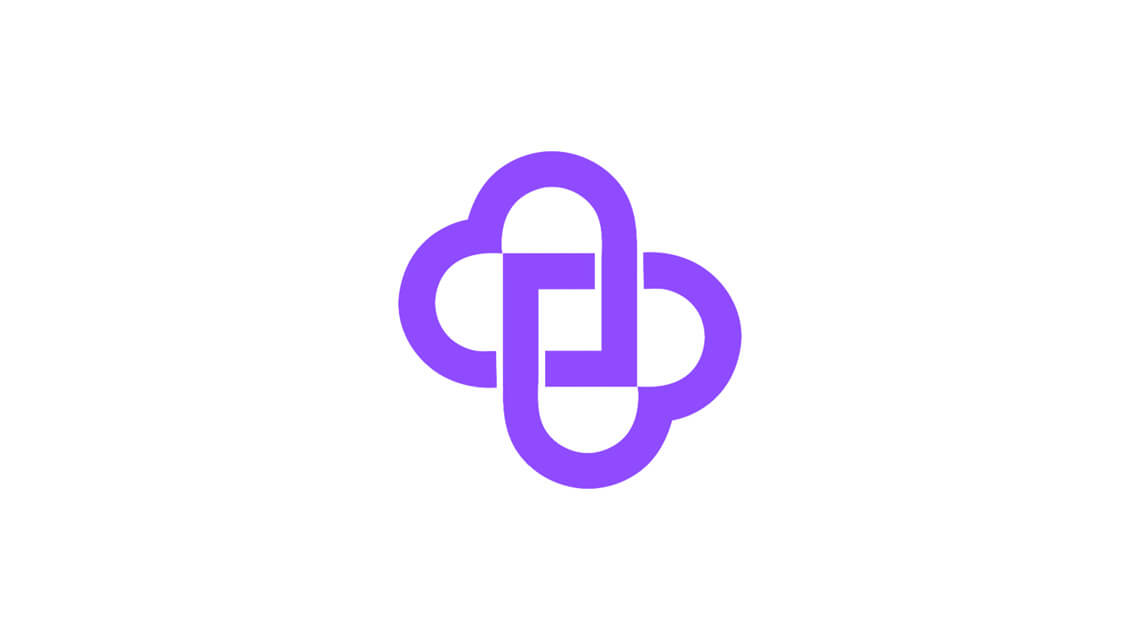
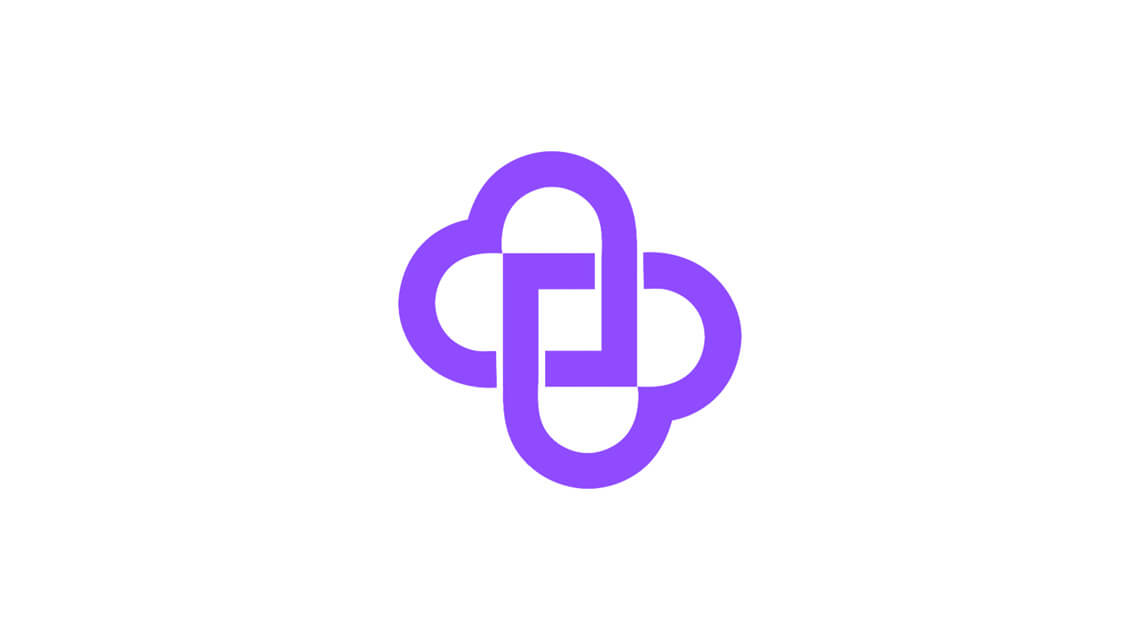